I recently wrote an article about removing Storyboards constraints programmatically. When you do that, you'll often also want to replace the removed constraints with new ones: you can do that - programmatically as well - by using the addConstraint method, which allows to set one or more constraints between any two UIView items.
Let's see some example by taking a standard UIButton item as the first object and its Parent View (or superview, as it is called by Objective-C) as the second one.
How to align an object to its ParentView Center X / Center Y
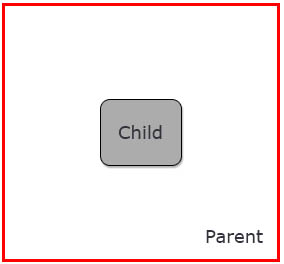
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 |
[self.btn.superview addConstraint:[NSLayoutConstraint constraintWithItem:self.btn.superview attribute:NSLayoutAttributeCenterX relatedBy:NSLayoutRelationEqual toItem:self.btn attribute:NSLayoutAttributeCenterX multiplier:1.0 constant:0.0]]; [self.btn.superview addConstraint:[NSLayoutConstraint constraintWithItem:self.btn.superview attribute:NSLayoutAttributeCenterY relatedBy:NSLayoutRelationEqual toItem:self.btn attribute:NSLayoutAttributeCenterY multiplier:1.0 constant:0.0]]; |
How to align an object to its ParentView Center X / Top Y
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 |
[self.btn.superview addConstraint:[NSLayoutConstraint constraintWithItem:self.btn.superview attribute:NSLayoutAttributeCenterX relatedBy:NSLayoutRelationEqual toItem:self.btn attribute:NSLayoutAttributeCenterX multiplier:1.0 constant:0.0]]; [self.btn.superview addConstraint:[NSLayoutConstraint constraintWithItem:self.btn.superview attribute:NSLayoutAttributeTop relatedBy:NSLayoutRelationEqual toItem:self.btn attribute:NSLayoutAttributeTop multiplier:1.0 constant:0.0]]; |
Align an object to its ParentView Left X / Top Y
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 |
[self.btn.superview addConstraint:[NSLayoutConstraint constraintWithItem:self.btn.superview attribute:NSLayoutAttributeLeft relatedBy:NSLayoutRelationEqual toItem:self.btn attribute:NSLayoutAttributeLeft multiplier:1.0 constant:0.0]]; [self.btn.superview addConstraint:[NSLayoutConstraint constraintWithItem:self.btn.superview attribute:NSLayoutAttributeTop relatedBy:NSLayoutRelationEqual toItem:self.btn attribute:NSLayoutAttributeTop multiplier:1.0 constant:0.0]]; |
I think you got it.
Happy coding!